07 Apr 2016
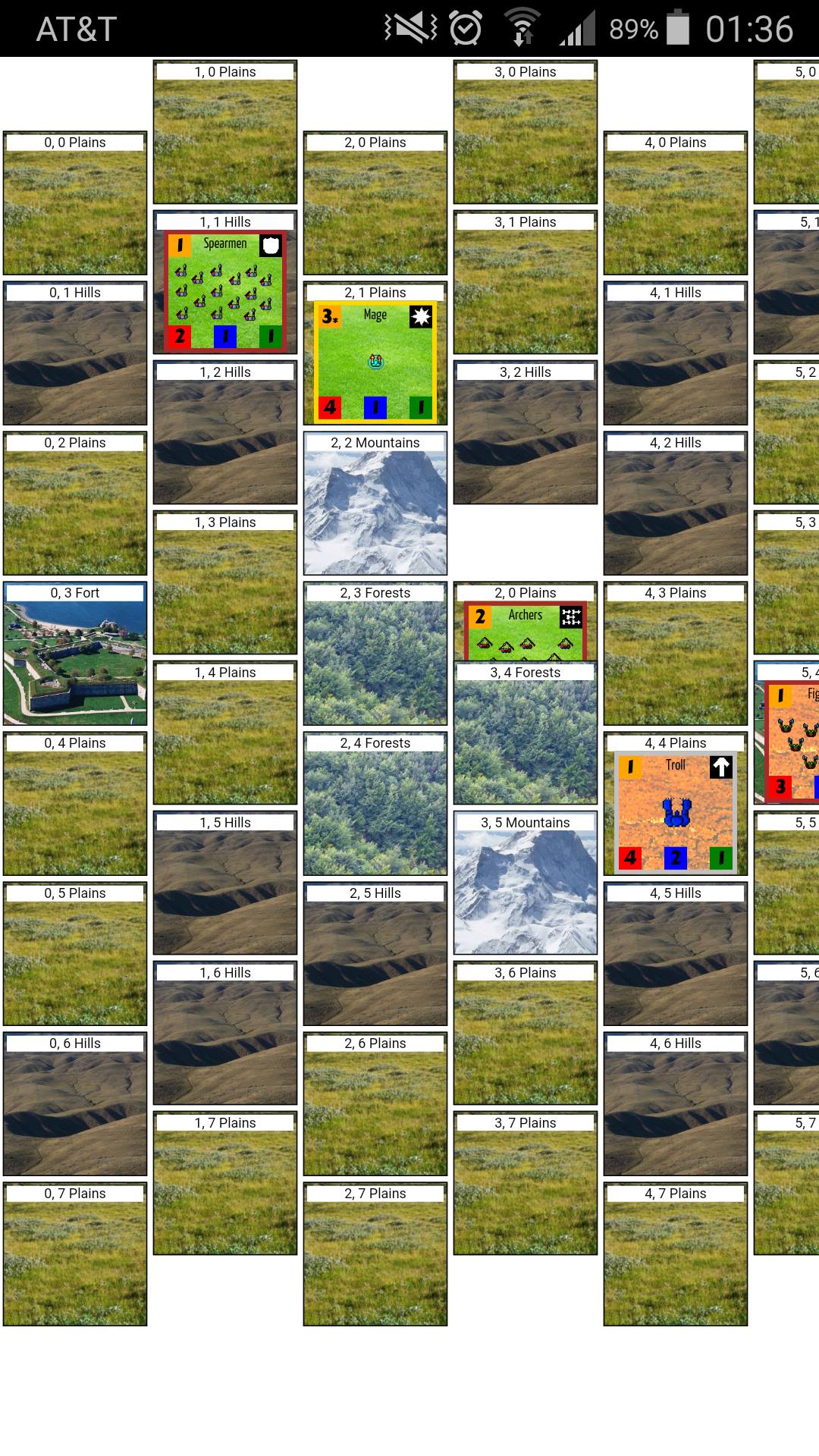
So I linked Kelly to the work I did last night and she sent me back this screenshot. That’s a nasty little gap in the middle there, and it’s a big problem. I clicked around myself and found that you can actually select several units at a time and have them possess the ~activeSpace~ class, which is not good since they will all move to the target space you pick and their empty spaces will be whatever the first one in the stack was sitting on, which throws off the display of the grid and makes more than one unit disappear.
if (activeGame.spaces[y][x].contains) {
$(this).addClass("activeSpace");
$("div#grid .gridSpace").each(function(){
$(this).removeClass("movable");
var targetClasses = $(this).attr("class").split(" ");
var targetX = targetClasses[2].charAt(3);
var targetY = targetClasses[1].charAt(3);
if (activeGame.spaces[y][x].canMoveTo(activeGame.spaces[targetY][targetX])) {
$(this).addClass("movable")
}
})
}
So what I need to do is take out the activeSpace class from whatever space has it from the get go.
if ($(".activeSpace")) {
$(".activeSpace").removeClass("activeSpace")
}
Nice and easy. Let’s see if it works.
Yeah, that did it. Nice.
06 Apr 2016
If you visit the testGrid you’ll see that you can now click on dudes and move them around at will. There isn’t currently anything preventing you from moving around all over the damn place and the thing is faction agnostic at this point, so it doesn’t have any attacking functions or anything, but it’s a pretty good start. Very happy, kind of tired.
The separate .render() function is important to how this works, because it replaces the HTML of the updated spaces with a fresh render of each space. Since the transfer of the Unit object occurs before the render takes place, it’s an adequate representation, and it should transfer clean with whatever temporary effects there are in play.
Right now the movability of the space is only represented in the rendered page and has no analog in the actual object code, which I think works fine. I suppose if I wanted to present the game as like an ASCII telnet thing I’d want to transfer that functionality into something a little more hard-coded, but I suppose it’d basically return true or false in reaction to like a “3,0 to 2,0” kind of query. It wouldn’t be that hard to do.
A telnet client would actually be a fun way to make this thing multiplayer without having to worry about handshaking or anything like that. How would you do a grid in ASCII?
_ _ _
/S\_/W\_/ \
\_/O\_/L\_/
/ \_/ \_/ \
\_/ \_/ \_/
Yeah I guess that would work fine. You’d want to pop it up a bit and have a little more room for characters so that you could show more information but no biggie.
03 Apr 2016
I’ve got a function up and running that returns a two dimensional array with space objects that can contain a unit. It can pass the unit to the renderUnitCard
function and can be easily traversed. Very satisfying.
["......",
"--..--",
".-^--.",
"=.##..",
"..##.#",
".--^-.",
"--..--",
"......"]
The Space class doesn’t need to be any more complicated, it’s really just a container though it’s one that should have a prototype output method that returns itself as a string, complete with any contained units. The game only allows one real token on the space at any given time which simplifes things. The Token class is going to need a prototype method that returns a string <div> version of itself so that the css can render the unit on top of the card.
I have to find some good backgrounds for the spaces too.
I’m thinking about the option of turning the game into a grid instead of having hexes, just for simplicity of rendering, but then you’d either have four facing with no diagonal movement/attack or you’d have eight facing, both of which just feel so different than having six facings which is of course the world’s most perfect number for faces of a polygon.
Oh yeah and it makes the map from sexy ASCII drawings like the one on the top.
02 Apr 2016
Things have been going a bit slow because the whole family just got over being sick all at the same time. Haven’t had a lot of time to stay up late and work on stuff, plus I download Kerbal Space Program which is a fascinating and educational time sink. It’s amazing how many people are talking about rocket mechanics for no reason other than accomplishing goals in a make believe sandbox video game space program. So far I’ve killed a lot of kerbals and made some explosions, and had a couple of falling with style types of situations.
I’ve been thinking about how to handle map movement for my game. I think the first thing to do is to get a working demo where a virtual table top, with an array of objects representing the spaces, is shown on the screen, and a guy can move around on the map. Maybe with some terrain, maybe worry about the terrain later.
Nah, I should worry about the terrain now.
So it’s going to look something like this:
var map = [
[ {
x: 0,
y: 0,
terrain: "Plains",
contains: {
"spearmen 1": {
"name": "spearmen",
"tokens": [
hit, hit, entrenched
]
}
}
]
]
Or something like that. And then it goes through it and renders it on the screen. And then you can click on the guy and it shows you where he can move and then you can click on one of those spaces and he moves there. And you can’t click on spaces where he can’t move.
Attacking is more complicated because of range. I also have to decide if I want the player to be able to course out a whole turn’s worth of movement or if I want everyone to pop around one space at a time. Rivers require a coin flip to cross so you wouldn’t be able to plan a route across a river because you don’t know if the ford will be successful or not.
31 Mar 2016
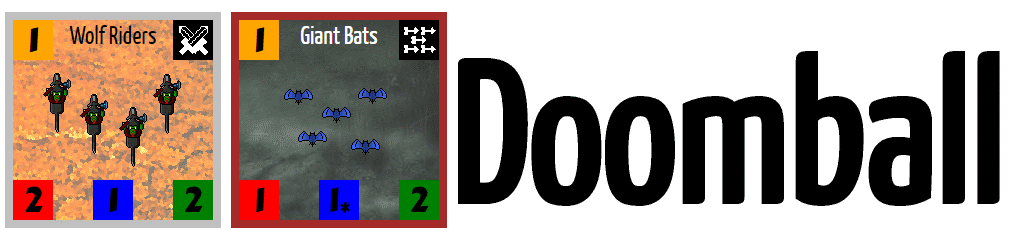
Kind of an excellent bit of prograss on the Doomball engine. I’ve got the unit renderer working, I made a way to work with the grid, and I’ve been putting some solid thought into the way to handle gameplay mechanics. Found a cool little game that does some similar things to what I’m thinking about, though it handles them in different ways. I think a big part of it is that I’ve been working with front end stuff so the simple way to handle things for me seems to be with jQuery and lots of div
classes and I think it could be handled more smoothly with pure JS and some canvas elements, but I don’t know how to use canvas elements honestly.
There’s a gallery of units up right now though that’s on the index.html file so if it changes that link will go to something different, and I think they’re all looking pretty good. I have art done for a few more races but I’ve gotten better at pixel art since I started so I kind of want to go back and revisit these old units. One benefit to this project, even if I don’t actually get it working as a video game, is that this is a way easier way to customize and revise these pieces than trying to keep up with the dramatic system of paint.net layers I was using before.
The grid system is a bit messy but the spacing is basically right. There was something weird that happened where the inline-block div elements that hold the units were dipping on the last line when it wrapped around the window, so I switched the display to regular block and just floated the things and that cleared it up. Didn’t really have to be inline-block anyway, there was nothing inline about the design.
I also wrote a kind of sloppy little function that checks for adjacency.
Space.prototype.isAdjacentTo = function(space){
if (this.x == space.x || this.x == space.x-1 || this.x == space.x+1) {
if (this.x % 2 == 0) {
if (this.y == space.y-1 || this.y == space.y) {
return true}
} else {
if (this.y == space.y || this.y == space.y+1) {
return true
}}}
return false
}
I wrote some grids down longhand and tried to figure out a good test to see if spaces were adjacent when you were dealing with hexagons and this is basically what I came up with. I thought it was too rough and I figured, since I didn’t really have any tests to run on it yet, I’d shelve it and see how it worked when I got to it but when I went looking for a good way to generate hex maps in html, I found this game and jumped into the source code. It basically uses the same algorithm to check adjacency, but it uses arrays of values to add or subtract from the values of the hex in question instead of a simple test like this. I’m not sure if it’s better or not. I guess you could use those values in more than one place, with the other guy’s example.
GridMath.neighbors = {
'even': [[-1, 0],[+1, 0],[0, -1],[0, +1],[-1, +1],[-1, -1]],
'odd': [[-1, 0],[+1, 0],[0, -1],[0, +1],[+1, +1],[+1, -1]]
};
GridMath.isNeighbor = function(x, y, x2, y2) {
var neighbors = y % 2 == 0 ? GridMath.neighbors.even : GridMath.neighbors.odd;
for (var i = 0; i < neighbors.length; i++) {
if (x + neighbors[i][0] == x2 && y + neighbors[i][1] == y2) {
return true
}
}
return false
};
See? Basically the same thing.