27 Mar 2016
Unit Generator
var Unit = function(unit) {
this.race = unit.race; this.name = unit.name;
if (unit.level) {this.level = unit.level}
else {this.level = 1}
this.range = setStat(unit, "range");
this.attack = setStat(unit, "attack");
this.defense = setStat(unit, "defense");
this.move = setStat(unit, "move");
if (unit.special) {this.special = unit.special}
this.tokens = [];
this.graphic = unit.graphic;
}
This is what I’m working with so far. setStat() is a simple utility function that sets a default stat and handles the availability of special characteristics. I think there’s a little bit more logic in here I could rip out but I can roll with this so far. Having defaults values (1 range, 2 attack, 1 defense, and 1 move) means that I can create unit definitions that simply state how they differ from the default instead of typing out each characteristic every time. That feels programmatic. It feels like a good solution.
Human Units
var humanUnits = {
"spearmen": {
"race": "human",
"name": "Spearmen",
"special": "Steadfast",
"graphic": "./images/spearmen.png"
},
"archers": {
"race": "human",
"name": "Archers",
"special": "Pinning",
"graphic": "./images/archers.png",
"range": {
"value": 2
},
"move": {
"special": true
}
},
"horsemen": {
"race": "human",
"name": "Horsemen",
"special": "Overrun",
"graphic": "./images/horsemen.png",
"defense": {
"special": true
},
"move": {
"value": 2
}
},
...
There’s four more like this, some more or less complicated, works out fine. I have another function that makes HTML strings that display these as the blocks I previewed in the last post, feels good. Have to throw together some transparent unit graphics and then get started on the next army I think - having functions that can display each faction with nothing more than a change in JSON archive is central to the design concept I’m working with here.
What’s next
-
I need to make sure that it changes the background image based on the faction. In the original design for the game each faction had a specific and different background image. I don’t know if it’s something I’m going to stick with but it’s something I want to at least work into the spec. I’m thinking each will have a specific class to throw onto the divs with a different background image - .unit-human, .unit-orc, .unit-hive, that sort of thing.
-
I’m still thinking about how to display these suckers responsively and how to give them a nice hex grid to get thrown onto. I think I can set up the actual display on the board with some judicious div use and a .staggered class that pops everything over 100 pixels. Getting the hexes to lock into each other will be tough, though. Have to figure out the right size to make them so that they can have the pieces on top and you can still see what the space is underneath. Each is going to have to be identifiable from its margins.
-
Haven’t even started on the game logic yet.
-
The tokens could be part of the tile generation. Throw some conditional .divs into the package and make sure they display on different places on the tile - the whole of the inside is claimable territory for tokens, but you wouldn’t want them to overlap by any means.
25 Mar 2016
I whipped this up
Spearmen
1
S
2
1
1
Frankly, it’s ugly as sin. The colors are HTML generic, the styling is all done in-line for testing purposes, the graphics could be a little better - should I code in multiple instances of individual soldier graphics and plug them in? They’re all based on a universal sizing template per size so it should theoretically be a thing, but I don’t know if it’d be better than using these basic png transparencies for it. Need to make sure the text can display dynamically so it’s visible on whatever size screen is looking at it, too. Do I want to set this up to be responsive from the get go or would I be better off keeping it simple at first and revising it later? Or never, and just making it for desktop? Everyone’s on phones these days, probably best to make it responsive, but it’s going to be awfule finicky to position in the first place.
As a proof of concept this is an ugly success.
24 Mar 2016
This game used to be called Artifact War
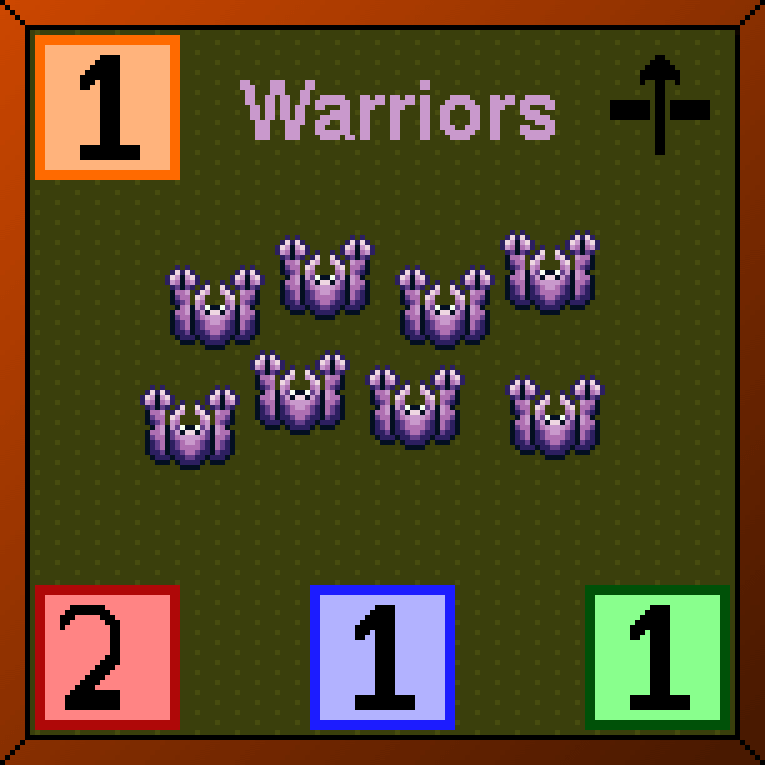
I made a board game last year, loosely based on American football, where two armies of fantasy races try to snag an artifact in the middle and run it past the opponent’s side of the board. I wrote up some rules, I wrote down some pseudocode logic because I wanted to turn it into a computer game, I made up some nine different races and hand drew the armies for them, I made custom pixel art to represent the pieces, I drew up templates and started researching how to be better at pixel art so that I could make it look better - I guess I kind of got into it.
Then I stepped away.
I haven’t sat down with this project for like a year, it was a fun one too. Now I think I’m going to try to leave my dream and turn my weird little board game into a computer game.
Tonight I started thinking about the constructors I’m going to need.
var Unit = function() {
this.race = "string";
this.name = "string";
this.id = 0;
this.level = 1;
this.range = {
"value": 1,
"special": false
};
this.attack = {
"value": 2,
"special": false
};
this.defense = {
"value": 1,
"special": false
};
this.move = {
"value": 1,
"special": false
};
this.special = "string";
this.tokens = [];
this.url = "string"
}
// Special abilities: Steadfast, Pinning, Overrun, Armor Piercing, Charging, Explosive, Cowardly, Leadership
This is going to have to be some kind of construction function, possibly taking an object as an argument and filling in default values if not specified, so parts will have to be replaced with more useful defaults. This might be a good place to add some prototype actions to let the characters move on the field.
var Space = function(){
this.x = 0;
this.y = 0;
this.terrain = "Plains"
}
I’m probably going to want to structure this so that it can be used in a gameboard constructing function, so the arguments are going to have to be like (x, y, terrain) and then the terrain will probably be a one letter thing so that I can specify maps pretty easily.
var Token = function(){
this.name = "string";
this.unique = true;
this.url = "string"
}
Was considering adding an x, y locator object and an owner object but I don’t know now, since I’m probably just going to push tokens into an array on the individual units anyway. Seems like the easier way to do it. Maybe add a prototype .effect() function that checks the string and then applies the appropriate effect to the character.
A lot is going to have to happen in the game logic, though, with various functions checking for the presence of certain tokens and then applying different effects as a matter of course. Some tokens will also be looking for the presence of other tokens, and I’m not sure how to do that.
23 Mar 2016
The Challenge
One of the early front-end challenges in the freeCodeCamp curriculum is to make a little app that gives you a random quote when you click a button. You’re also supposed to make it so that you can tweet the quote if it’s a good one, but that was optional when I did the challenge. I think I’m going to have to go back and add that on though because they took out optional User Stories and made them all mandatory, I guess we’ll see.
The Archive
I started by looking for a solid API to provide random quotes and I found quite a mess. Lots of websites that wanted to be like “you can only do so many quotes” or gave instructions I didn’t really get at the time, so I decided to skip that mess and do it myself. I split it up into three parts - the first part was a simple array containing the quotes I’d picked out as what were coincidentally JSON objects. I don’t think I totally understood JSON objects at the time.
{'id': 0, 'quote': 'string', 'author': 'string', 'source': 'string'}
So that worked out pretty well. I could generate a random number between 1 and the number of quotes and use that to snag the quote I wanted to render on the page.
The Collector
Once I started grabbing quotes online and throwing them into the page, though, I realized that it was tedious and I hated it, so I threw together a quick page that gave me a form I could put the info into to get a nicely formatted object to copy-paste into the database. Obviously a full stack program would submit it directly, but this is Codepen so it’s all front end stuff.
See the Pen QuoteForm by James Beardsley (@beardsley-james) on CodePen.
$('#id').val(quotes.length);
This part sets the ID number as the next one higher than the highest one in use so I don’t have to figure it out manually.
$('#quoteGen').on('click', function(){
$('#result').text('{id: ' + $('#id').val() + ', quote: "' + $('#quote').val() + '", author: "' + $('#author').val() + '", source: "' + $('#source').val() + '"\} ');
})
This is the meat, this snags values out of all the form sections and tosses them together into a juicy javascript object. I think now I would probably pull in some variables to gussy it up a bit but honestly this is so clean and functional I don’t think it’d be an improvement. Also note the use of single quotes - I like double quotes usually since I’m an American but all the quotes I was inputting had double quotes too, so I had to use single quotes to not break the scripting.
for (i = quotes.length - 1; i > 0; i--) {
document.write("</br><p>ID: " + quotes[i].id);
document.write("</br>Quote: " + quotes[i].quote);
document.write("</br>Author: " + quotes[i].author);
document.write("</br>Source: " + quotes[i].source)
}
And this part prints all the existing quotes onto the page when it loads, which is a nice feature. It’s not totally necessary I suppose, but it doesn’t hurt.
I didn’t think to throw everything into the mandatory $(document).ready()
jQuery handler, but it doesn’t seem to have hurt the functionality any so no worries on that front.
The Product
Finally I threw together the actual random quote generator which wasn’t really as inspired as the pseudo back end I don’t think.
See the Pen Random Quote Generator by James Beardsley (@beardsley-james) on CodePen.
I made one in yellow.
See the Pen RQG Candy Apple Green by James Beardsley (@beardsley-james) on CodePen.
And I made this one called candy apple green for some reason. I don’t really know why. It doesn’t have any actual green on it but that’s what it’s called. I really don’t remember where the name came from.
var previous;
$(document).ready(function() {
$('#button').on('click', function() {
var i = Math.floor((Math.random() * quotes.length) + 1);
if (i === previous) {
i++
};
if (i > quotes.length) {
i = 1
};
previous = i;
$('#quote').html(quotes[i].quote);
$('#author').text(quotes[i].author);
$('#source').text(quotes[i].source)
})
});
One of the big things that bothered me about the original version of this was that sometime you would click on it and it would just show you the same quote as the one you already had. No big deal if there were a lot of quotes, since the chances of getting a duplicate were pretty low, but my enthusiasm for digging up appropriately pretentious quotes flagged pretty quickly. Nice and easy, though, just saves whatever the ID of the current quote is and adds one if it’s a duplicate. Then I ended up with blank pages when it ran into the ceiling, so I made it reset back to 1 if it hit the end. Other than that it’s just filling in some variables and throwing them onto the page.
I put this sucker together like a year ago but I think it holds up pretty well. Someone in the freeCodeCamp chatroom yesterday said they liked how clean the javascript was and so do I. It’s a good indication of my proclivity for modulization, which I hope to explore going forward.
22 Mar 2016
I’ve been play Smite lately. A few years ago I saw my brother-in-law playing a Warcraft III mod where teams of heroes smacked up mobs of npcs and each other on a three lane map and I didn’t really think it was going to end up being a subgenre, but here we are seven years later and people are playing dressed up versions of that game for a million dollar while I didn’t have a good gaming PC.
I found the new Tribes game on a free games list and when it was downloading this other game they made called Smite where gods smack each other up, and mobs of npcs too, and I decided to try it since I have a nice new computer. It’s pretty good.
So far I’ve lost a lot of matches and gotten a few satisfying kills. My boy is Poseidon, he can make a kraken come up and smack people up for him which is pretty great. His abilities are tough to aim sometimes but it’s no big deal. I miss sometimes, I think if I hit every time I’d probably have more kills.
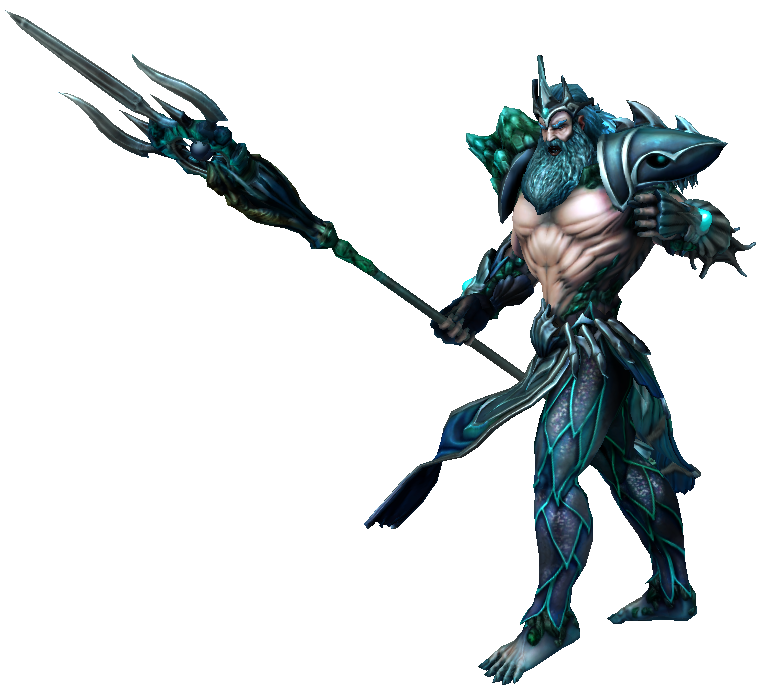
He gets faster when he hits people, though. I watched a video that said to hang back and throw shade at dudes from far away until you get your ultimate up and then try to get a hero kill and it seemed to work. I’ve been playing with auto items for streamlining reasons for a bit but I’ve started picking my own weapons and it’s working alright.
I switched over to Ra because I would get a bunch of gems for mastering a character and he was pretty sick, but now I’m messing around with a big thug ice giant that breathes ice and also can shoot ice spikes out of his back. Good times.