21 Mar 2016
Like, I’m sure, many of my readers, I frequently read 300 page rulebooks for games I never intend on playing simply for the pleasure of studying irrelevant minutiae. In the course of reading the latest version of Warhammer Fantasy Roleplay, a really gritty and fun roleplaying game for people that have a keen dislike for the characters they’re roleplaying, I found myself wondering how some of the combat mechanics might play out if they were automated.
At this point I was basically just messing around on Codepen. I came up with this:
See the Pen Warhammer Adventure by James Beardsley (@beardsley-james) on CodePen.
Basically you can click on a couple buttons, come up with some semi-randomized Warhammer Fantasy critters, and then make them fight to the death for fun, with a nice little adventure read out that tells you how hard they’re hitting each other.
Now there are some serious balance issues with this “game”, specifically that there’s this whole system of avoiding combat that only applies to opponents that are using ranged weapons, which means nine times out of ten your orc buddy is going to stomp whatever poor halfling archer you’re sending his way.
I had to make some tough choices about what kinds of characters I was going to include, too, and I haven’t even put in a magical system or any kind of way of keeping a range value between the character to make bows and arrows more effective.
I’d like to put in some kind of ammo counter too, so that guns are more of a thing. Tighten up a bit. It’s tough to feel that motivated to, though, since as far as I can tell the major consumer category for this program consists of me and I’m not even that interested in running it a bunch of times. Fun exercise, though, and it’s really the first time I tried to stretch my legs out with object oriented programming concepts.
The latest version is here, which I moved over from Codepen when I decided to host on Github for now. The main thing I did in the move was separate the parts of the Javascript code bank into their own separate files which isn’t at all best practice at this point, and make the form to pick characters a bit more robust and interesting.
I also added an ability for the game to store and serve pre-generated characters types, which is something I might revisit when I start hooking some of these things up to backend software.
19 Mar 2016
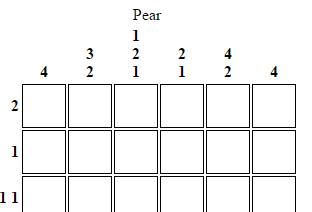
The Nonogram generator is my biggest project to date, and the one I’ve got the most significant plans for.
Last year I started playing a game called Griddlers Plus on my telephone, it’s a pretty simple Nonogram game with colors and triangles spaces which is pretty great. I have a couple of strategies I’ve figured out for solving the puzzles, and I was looking into transferring them into a javascript program I would use to solve the puzzles for me or at least give me a head start.
In the process of looking into doing so, I realized quickly that I would essentially have to reinvent the game in order to program the solving algorithm, and I started working on an engine to render the grid as a usable artifact on Codepen.
See the Pen Nonogram by James Beardsley (@beardsley-james) on CodePen.
I ran into a couple of headaches and realized I didn’t necessarily have the capacity to work this out on Codepen. I think the main problem was that I thought I should be able to have a function that could traverse both columns and rows to output the binary value representing activated cells. I started working on other projects and got more comfortable using github to host my online content.
At this point I began work on the next iteration of the nonogram software, mostly working on setting up the form that would be used to input the values and get it set up. I started working with jQuery and worked out the basic structure I wanted on notepaper at work before I took it home and started working with it.
function generateBinaryString(element){};
function generateClue(binaryString){};
function generateClassFromValue(val){
switch (val){
case "0":
return "white";
break;
case "1":
return "black";
break;
case "2":
return "flag"
}
};
I was also reading Clean Code by Robert Martin at the time so I was thinking a lot about how to name functions and using little functions to do every damn kind of thing.
I ran into basically the same problem, though, especially when I tried accessing classes in freshly made DOM nodules with jQuery. I decided to try something a little different and looked up how to set up a table using straight javascript DOM manipulation.
function tableMaker(tableId, puzzle) {
var table = document.getElementById(tableId);
table.innerHTML = ("");
var tbody = document.createElement('tbody');
var width = puzzle.width;
var height = puzzle.height;
table.appendChild(tbody);
for (var i = 0; i <= height-1; i++) {
tbody.insertRow(i);
for (var j = 0; j <= width-1; j++) {
tbody.rows[i].insertCell(j);
tbody.rows[i].cells[j].addClassName("row" + i);
tbody.rows[i].cells[j].addClassName("cell" + j);
};
}
tbody.insertRow(0);
for (var j = 0; j <= width-1; j++) {
var th = document.createElement('th');
th.addClassName("cell" + j)
tbody.rows[0].appendChild(th);
}
tbody.rows[0].insertBefore(
document.createElement('td'), tbody.rows[0].cells[0]
).addClassName("empty");
for (var i = 1; i <= height; i++) {
var th = document.createElement('th');
th.addClassName("row" + (i - 1))
tbody.rows[i].insertBefore(th, tbody.rows[i].cells[0])
}
table.createCaption().innerHTML = puzzle.name;
return true;
}
Of course this didn’t work out right away and I had to tweak stuff but suddenly I had an appropriate DOM object to work with, consistent across instances importantly, since prepending the table headers in front of the rows was one of the things throwing me off with jQuery. What I originally referred to as “TableMaker” became Griddler 2, and I think is the most usable bit of programming I’ve done to date. You can go in, you can make objects that could theoretically be exported into the program as solvable puzzles, you can solve puzzles and it tells you when you win. I think the clue verification is still a bit wonky, and there’s nowhere to input your created puzzles to make them usable, but it’s a start.
The plan going forward is to put in some kind of backend so that people can use their Facebook profiles or whatever to jump on and save their puzzles. Nothing big, it’s really a practice project, but it’s nice to have something I kind of ran at and conquered of my own volition.